1. Tutorials
1.1. Parsing and Stitching Data from Opera Phenix
First you need to export your data from Harmony and rename the path to eliminate any spaces in the name. Then you can run the following script to parse and stitch your data.
example script for parsing and stitching phenix data
#import relevant libraries
import os
from sparcstools.parse import parse_phenix
from sparcstools.stitch import generate_stitched
#parse image data
path = "path to exported harmony project without any spaces"
parse_phenix(path, flatfield_exported = True, export_as_symlink = True) #export as symlink true enabled for better speed and to not duplicate data, set to False if you want to work with hardcopies or plan on accessing the data from multiple OS
#define important information for your slide that you want to stitch
# the code below needs to be run for each slide contained in the imaging experiment!
# Can be put into a loop for example to automate this or also can be subset to seperate
# jobs when running on a HPC
input_dir = os.path.join(path, "parsed_images")
slidename = "Slide1"
outdir = os.path.join(path, "stitched", slidename)
overlap = 0.1 #adjust in case your data was aquired with another overlap
#define parameters to find correct slide in experiment folder
overlap = 0.1 #adjust in case your data was aquired with another overlap
row = str(2).zfill(2) #specify the row of the well you want to stitch
well = str(4).zfill(2) #specifc the well number you wish to stitch
zstack_value = str(1).zfill(3) #specify the zstack you want to stitch. for multiple zstacks please make a loop and iterate through each of them.
timepoint = str(1).zfill(3) #specifz the timepoint you wish to stitch
#define on which channel should be stitched
stitching_channel = "Alexa647"
output_filetype = [".tif", "ome.zarr"] #one of .tif, .ome.tif, .ome.zarr (can pass several if you want to generate all filetypes)
#adjust cropping parameter
crop = {'top':0, 'bottom':0, 'left':0, 'right':0} #this does no cropping
#crop = {'top':72, 'bottom':52, 'left':48, 'right':58} #this is good default values for an entire PPS slides with cell culture samples imaged with the SPARCS protocol
#create output directory if it does not exist
if not os.path.exists(outdir):
os.makedirs(outdir)
#define pattern to recognize which slide should be stitched
#remember to adjust the zstack value if you aquired zstacks and want to stitch a speciifc one in the parameters above
#you can define different rescale ranges for each channel if you want to or alternatively use the same range for all channels
#same range for all channels
rescale_range = (1, 99)
#custom for each channel
rescale_range = {"Alexa647":(1, 98), "DAPI":(1, 99), "mCherry":(1, 99)} #channels need to be named as is the case in the parsed folder
pattern = f"Timepoint{timepoint}_Row{row}_Well{well}_{{channel}}_zstack{zstack_value}_r{{row:03}}_c{{col:03}}.tif"
generate_stitched(input_dir,
slidename,
pattern,
outdir,
overlap,
crop = crop ,
stitching_channel = stitching_channel,
filetype = output_filetype,
plot_QC = True,
do_intensity_rescale = True,
rescale_range = rescale_range)
1.1.1. Generated output
The stitching script will generate the following files:
.
├── QC_edge_quality.pdf
├── QC_edge_scatter.pdf
├── stitching_test.XML
├── stitching_test.ome.zarr
├── stitching_test_Alexa488.tif
├── stitching_test_DAPI.tif
├── stitching_test_mCherry.tif
└── stitching_test_tile_positions.tsv
FileName |
Contents |
---|---|
QC_edge_quality.pdf |
This plot shows which tiles are connected with one another. |
QC_edge_scatter.pdf |
Plots the alignment quality against each other |
stitching_test.XML |
contains the required input for reading the stitched files into BIAS |
<slidename>.ome.zarr |
stitched image containing all channels in the ome.zarr format, optimal for viewing in napari |
<slidename>_<channel_name>.tif |
stitched image for the given channel |
stitching_test_tile_positions.tsv |
coordinate position of where each tile is located |
1.1.2. Example Results
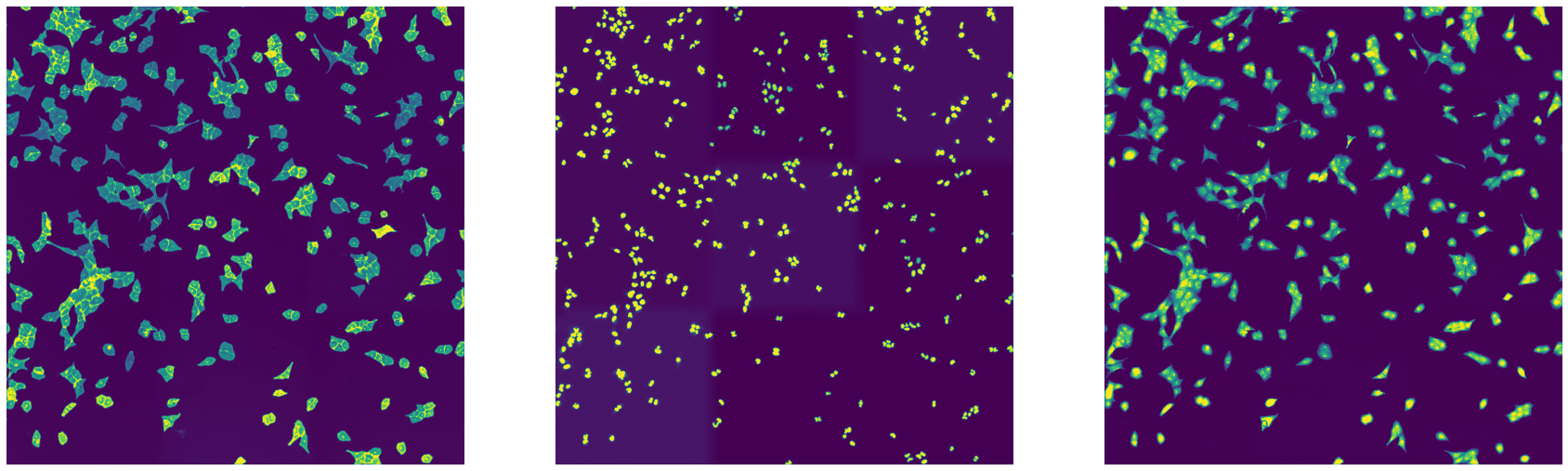